Essential Python Libraries for Machine Learning and Data Science
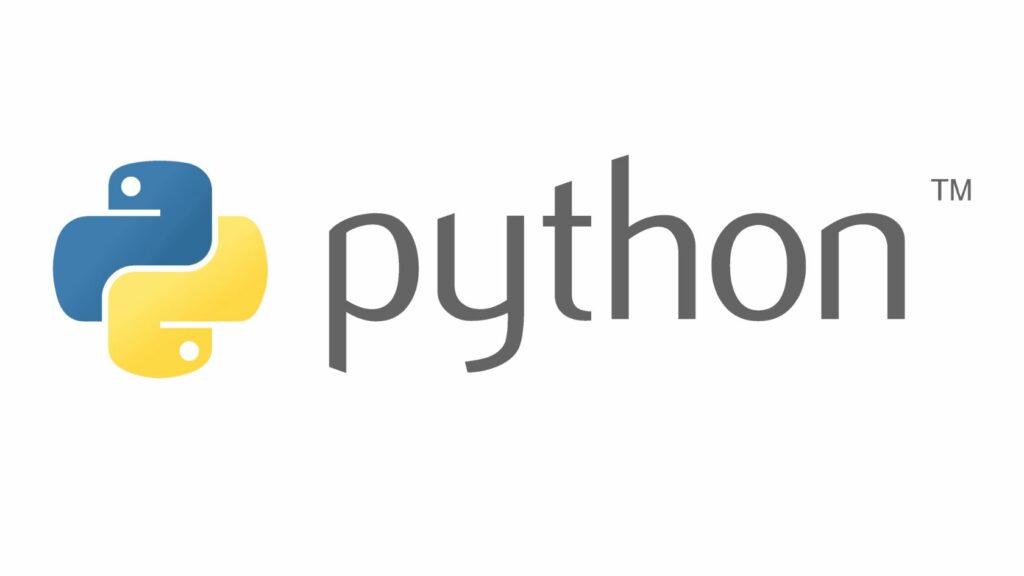
Python is the de facto programming language of the AI community. It’s easy to learn, and writing programs is a snap once you are proficient.
Thanks in large part to its open source libraries, Python users can manipulate data, prototype models, analyze outputs, and perform many other machine learning and data science tasks.Â
This post is aimed at people just beginning to use Python for AI as well as those who have experience but have questions about what to learn next. We’ll take a moment from time to time to fill in beginners about basic terms and concepts. If you’re already familiar with them, we encourage you to skip over the more elementary material and read on for perspective on finer points like graph execution and eager executions. This post will explain the most essential Python libraries and packages for AI, explain how to use them, and go through their strengths and weaknesses.
The most widely used Python Libraries for AI and ML
Adding the right mix of libraries to your development environment is crucial. The following packages and libraries are vital for most AI developers. All of them are freely available as open source distros.

Scikit-learn: If you need to do machine learning
What is it: Scikit-learn is a Python library for implementing machine learning algorithms.
Background: A developer named David Cournapeau originally released scikit-learn as a student in 2007. The open source community quickly adopted it and has updated it numerous times over the years.
Features: The packages in Scikit-learn focus on modeling data.
- Scikit-learn includes every core machine learning algorithm, among them vector machines, random forests, gradient boosting, k-means clustering, and DBSCAN.
- It was designed to work seamlessly with NumPy and SciPy (both described below) for data cleaning, preparation, and calculation.
- It has modules for loading data as well as splitting it into training and test sets.
- It supports feature extraction for text and image data.
Best for: Scikit-learn is a must-have for anybody working in machine learning. It is considered one of the best libraries available if you need to implement algorithms for classification, regression, clustering, model selection, and more.
Downsides: Scikit-learn was built before deep learning took off. While it works great for core machine learning and data science jobs, if you are building neural nets you’ll need either TensorFlow or Pytorch (below).
Best place to learn: Machine Learning in Python with Scikit-Learn from Data School. (Note: Scikit-learn is one of the easiest Python libraries to learn. Once you are proficient in Python itself, Scikit-learn is a snap.)

NumPy: If you need to crunch numbers
What is it: NumPy is a Python package for working with arrays, or large collections of homogenous data. You can think of an array like a spreadsheet, where numbers are stored in columns and rows.
Background: Python wasn’t originally intended for numerical computation when it was launched in 1991. Still, its ease of use caught the scientific community’s attention early on. Over the years, the open source community developed a succession of packages for numerical computing. In 2005, developer Travis Oliphant combined over a decade’s worth of open source developments into a single library for numerical computation, which he called NumPy.
Features: The core feature of NumPy is support for arrays, which allows you to quickly process and manipulate large collections of data.
- Arrays in NumPy can be n-dimensional. This means the data can be a single column of numbers, or many columns and rows of numbers.
- NumPy has modules for performing some linear algebra functions.
- It also has modules for graphing and plotting numerical arrays.
- Data in NumPy arrays is homogenous, which means it must all be defined as the same type (numbers, strings, Boolean values, etc.). This means data gets processed efficiently.
Best for: Manipulating and processing data for more advanced data science or machine learning operations. If you are crunching numbers, you need NumPy.
Downsides: Because NumPy arrays are homogeneous, they are a bad fit for mixed data. You are better off using Python lists. Also, NumPy’s performance tends to drop off when working with more than 500,000 columns.
Best place to learn: Linear Regression with NumPy and Python from Coursera.

Pandas: If you need to manipulate data
What is it: Pandas is a package for simultaneously working with different types of labeled data. You’d use it, for example, if you need to analyze a CSV file containing numerical, alphabetical, and string data.
Background: Wes McKinney released Pandas in 2008. It builds on NumPy (and, in fact, you must have NumPy installed to use Pandas) and extends that package to work with heterogeneous data.
Features: The core feature of Pandas is its variety of data structures, which let users perform an assortment of analysis operations.
- Pandas has a variety of modules for data manipulation, including reshape, join, merge, and pivot.
- Pandas has data visualization capabilities.
- Users can perform mathematical operations including calculus and statistics without calling on outside libraries.
- It has modules that help you work around missing data.
Best for: Data analysis.
Downsides: Switching between vanilla Python and Pandas can be confusing, as the latter has a slightly more complex syntax. Pandas also has a steep learning curve. These factors, combined with poor documentation, can make it difficult to pick up.
Best place to learn: Introduction to Pandas from DeepLearning.AI.

SciPy: If you need to do math for data science
What is it: SciPy is a Python library for scientific computing. It contains packages and modules for performing calculations that help scientists conduct or analyze experiments.
Background: In the late 1990s and early 2000s, the Python open source community began working on a collection of tools to meet the needs of the scientific community. In 2001, they released these tools as SciPy. The community remains active and is always updating and adding new features.
Features: SciPy’s packages comprise a complete toolkit of mathematical techniques from calculus, linear algebra, statistics, probabilities, and more.
- Some of its most popular packages for data scientists are for interpolation, K-means testing, numerical integration, Fourier transforms, orthogonal distance regression, and optimization.
- SciPy also includes packages for image processing and signal processing.
- The Weave feature allows users to write code in C/C++ within Python.
Best for: SciPy is a data scientist’s best friend.
Downsides: Some users have found SciPy’s documentation lacking and critique several of its packages as inferior to similar packages found in MatLab.
Best place to learn: SciPy Programming by Ahmad Bazzi.
If you need to do machine learning: TensorFlow vs. PyTorch
TensorFlow and PyTorch perform the same essential tasks related to deep learning: They make it easy to acquire data, train models, and generate predictions. From face recognition to large language models, many neural networks are coded using either TensorFlow or PyTorch. The libraries were once markedly different, both in the front and back-end. Over time, they converged around the same set of best practices.
Nonetheless, debate is ongoing within the AI community about which is best. TensorFlow, released in 2015, was the first on the scene. It dominates in commercial AI and product development, but many users complain about its complexity.
PyTorch, released in 2016, is widely considered to be both easier to learn and faster to implement. It is a favorite among academics and is steadily gaining popularity in industry. However, it is known to struggle at scaling.
Which to choose?
TensorFlow is still the dominant deep learning library in industry. This is partly due to inertia, and partly due to the fact that TensorFlow is better than PyTorch at handling large projects and complex workflows. Its ability to handle AI products that are scaled for commercial deployment makes it a favorite for product development.
If you are just jumping into deep learning and want to focus on building and prototyping models quickly, PyTorch is probably the better bet. Be aware that you may have to learn TensorFlow one day depending on your job requirements and company tech (especially if your dream job is at Google, home of TensorFlow).
Learn more about the pros and cons of both libraries below.
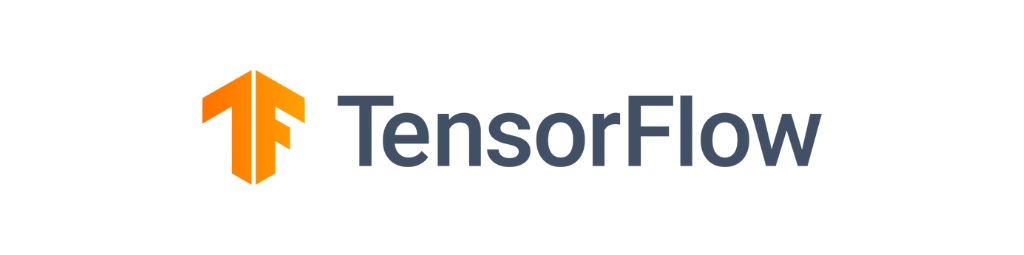
TensorFlow
What is it? TensorFlow is an end-to-end open source library for developing, training, and deploying deep learning models.
Background: TensorFlow was originally released in 2015 by Google Brain. Originally, its front end wasn’t user friendly, and it had redundant APIs that made building and implementing models cumbersome. Many of these issues have been resolved over time with updates, as well as by integrating Keras (see below) as the default front end.
Features: TensorFlow has numerous packages for building deep learning models and scaling them for commercial deployment.
- TensorFlow users can call upon the hundreds of pre-trained models in the Dev Hub and Model Garden. The Dev Hub contains plug-and-play models while the Model Garden is intended for more advanced users who are comfortable making customizations.
- It is efficient in its use of memory, making it possible to train multiple neural networks in parallel.
- TensorFlow applications can run on a wide variety of hardware systems, including CPUs, GPUs, TPUs, and more.
- TensorFlow Lite is optimized for mobile and embedded machine learning models.
- Users can freely upload and share their machine learning experiments on Tensorboard.dev.
Best for: Building production-ready deep learning models at scale.
Downsides: Some users still complain that the front-end is fairly complicated. You may also come across critiques that TensorFlow executes slowly. This is mostly a legacy complaint from TensorFlow 1.0, when it executed operations in graph mode by default. TensorFlow 2.0 defaults to eager execution mode.
Best place to learn: TensorFlow Developer Professional Certificate from DeepLearning.ai.
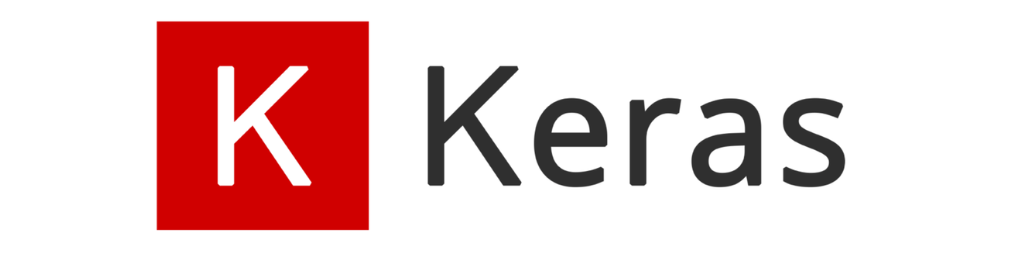
Keras:
What is it: Keras is a beginner-friendly toolkit for working with neural networks. It is the front-end interface for TensorFlow.
Background: Google engineer Francois Choillet released Keras in 2015 to act as an API for a number of deep learning libraries. As of 2020, Keras is exclusive to TensorFlow.
Features: Keras handles the high level tasks of building neural networks in TensorFlow, and as such contains fundamental modules like activation functions, layers, optimizers, and more.
- Keras supports vanilla neural networks, convolutional neural networks, and recurrent neural networks as well as utility layers including batch normalization, dropout, and pooling.
- It is designed to simplify coding deep neural networks.
Best for: Developing deep learning networks.
Downsides: It’s only available for TensorFlow users. If you use TensorFlow, you’re using Keras.
Best place to learn: Introduction to Deep Learning and Neural Networks with Keras from IBM.

PyTorch
What is it: PyTorch is Facebook AI Research Lab’s answer to TensorFlow. It is an open source, general-purpose library for machine learning and data science, specifically deep learning.
Background: Facebook released PyTorch in 2016 — a year after TensorFlow — and it quickly became popular with academics and other researchers who were interested in rapid prototyping. This was due to its streamlined front end and the fact that its default mode executes operations immediately (as opposed to adding them to a graph for later processing, as did TensorFlow).
Features: PyTorch has many features that are analogous those in TensorFlow. Indeed, in the years since they launched, each library has been updated to include the features that users like best about the other.
- PyTorch has its own libraries for pre-trained models. The PyTorch Hub is aimed at academic users who want to experiment with the model design, and the Ecosystem Tools contains pre-trained models.
- PyTorch is memory-efficient and accommodates training multiple models in parallel.
- It supports a variety of hardware types.
Best for: Rapid prototyping of deep learning models. Pytorch code runs quickly and efficiently.
Downsides: Some users report that PyTorch struggles with larger projects, big datasets, and complex workflows. Developers who build AI products to be deployed at scale may prefer TensorFlow.
Best place to learn:PyTorch tutorials from PyTorch.org.
Conclusion
The maturity of libraries for Python is one of the main reasons why it is so popular among the AI community. They make it easy to extend Python to tasks well beyond its original design. Once you have a firm grasp of the Python language and the libraries that pertain to your job, you’ll be able to build, train, and iterate on machine learning models for a wide range of applications.
Even with all its libraries, however, Python doesn’t excel at everything. For instance, if you are working on AI infrastructure you might need to learn C++; If you work in finance, you will probably need to learn R. To learn more about other AI programming languages and their uses, read our guide.
No matter what your AI goals are, the best thing to do is always keep learning!
Want more tips for building your AI career?